380
views
views
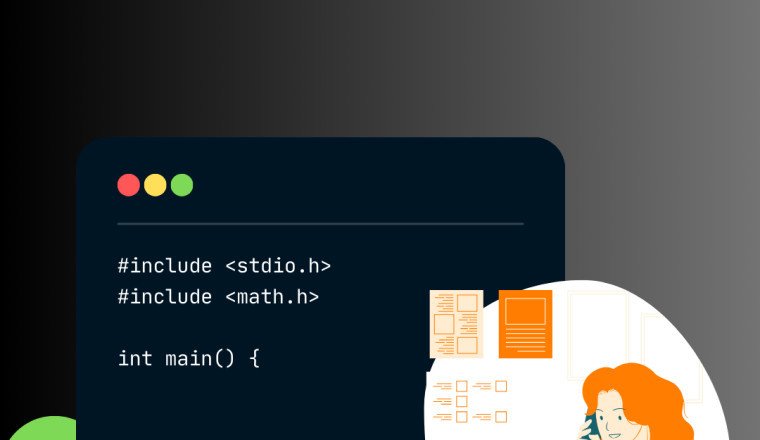
PROGRAMMING FOR PROBLEM SOLVING - Mohan Babu University - B.Tech Mentor
C Program to Find the Roots of a Quadratic Equation and to Calculate the Number of Illiterate Men and Women in a Town
a) Write a C program to find the roots of a quadratic equation.
b) In a town, the percentage of men is 52. The percentage of total literacy is 48 and the total percentage of literate men is 35 of the total population. Write a C program to find the total number of illiterate men and women if the population of the town is 7000.
a) This program takes the coefficients (a, b, and c) of the quadratic equation as input and calculates the roots.
#include
#include
int main() {
double a, b, c, discriminant, root1, root2;
printf("Enter the coefficients of the quadratic equation (a, b, c): ");
scanf("%lf %lf %lf", &a, &b, &c);
// Calculate the discriminant
discriminant = b * b - 4 * a * c;
// Check the nature of roots
if (discriminant > 0) {
// Real and distinct roots
root1 = (-b + sqrt(discriminant)) / (2 * a);
root2 = (-b - sqrt(discriminant)) / (2 * a);
printf("Roots are real and distinct: %.2lf and %.2lf\n", root1, root2);
} else if (discriminant == 0) {
// Real and equal roots
root1 = root2 = -b / (2 * a);
printf("Roots are real and equal: %.2lf\n", root1);
} else {
// Complex roots
double realPart = -b / (2 * a);
double imaginaryPart = sqrt(-discriminant) / (2 * a);
printf("Roots are complex: %.2lf + %.2lfi and %.2lf - %.2lfi\n", realPart, imaginaryPart, realPart, imaginaryPart);
}
return 0;
}
b) To find the total number of illiterate men and women in a town with a population of 7000, given the percentages of men, total literacy, and literate men, you can use the following C program:
#include
int main() {
int totalPopulation = 7000;
double percentageMen = 52.0;
double percentageTotalLiteracy = 48.0;
double percentageLiterateMen = 35.0;
// Calculate the number of men, literate men, and literate women
int numMen = (percentageMen / 100) * totalPopulation;
int numLiterateMen = (percentageLiterateMen / 100) * totalPopulation;
int numLiterateWomen = (percentageTotalLiteracy / 100) * totalPopulation - numLiterateMen;
// Calculate the number of illiterate men and women
int numIlliterateMen = numMen - numLiterateMen;
int numIlliterateWomen = totalPopulation - numMen - numLiterateWomen;
printf("Number of illiterate men: %d\n", numIlliterateMen);
printf("Number of illiterate women: %d\n", numIlliterateWomen);
return 0;
}
Comments
0 comment